If we want to supply any input (as argument to the main() method) to the program at the beginning of execution, we can use command line arguments.
Command-Line Arguments are the arguments that are supplied to the program, when it is called for execution.
When the program is ready to run, the arguments are provided along with the program’s name (in the console) using whitespace as the separator.
Syntax:
c:\ java ProgramName <space> Arg1 <space> Arg2
Example:
Program Name : Demo.java
class Demo
{
public static void main (String user_input[])
{
System.out.println(“First Argument is “+user_input[0]);
System.out.println(“Second Argument is “+user_input[1]);
}
}
Compile:
javac Demo.java
Run:
java Demo Coding Dots
Output:
First Argument is Coding
Second Argument is Dots
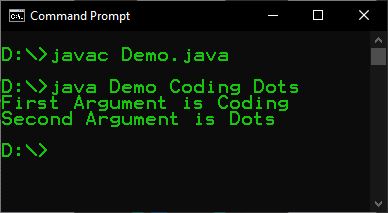
Explanation:
In the above example when we run our program with two command line arguments (given after the program name), they are stored in user_input array (i.e. an array of string objects) and then they are shown as output using println() method.
user_input[0] => Coding
user_input[1] => Dots
NOTE:
Command line arguments are string objects by default.
If we pass any number as command line argument, it is not treated as an integer (i.e. 1), it will be treated as string(i.e. “1”), and we have to convert it into an integer for performing numeric operations on it.
For converting a string to an integer, we can use the Wrapper class methods, e.g.
Integer.parseInt(user_input[0])
This method converts a string ( having numeric characters ) into an integer, i.e. from “100” to 100.